Customer engagement is the cornerstone of not only attracting but also nurturing and retaining a loyal customer base — a vital element for any business aiming for sustainable growth and success. However, to improve your customer engagement marketing, you need a dependable way of benchmarking your campaign performance. This is where customer engagement metrics come in.
In this article, we take a deep dive into customer engagement, shining a light on the top 8 metrics that are crucial for businesses to track. By mastering these metrics, you’ll gain a better understanding of your customers’ journey with your brand and strategically position yourself for growth and adaptability in a dynamic market.
Why Customer Engagement Metrics Matter
With customer expectations continually rising and the cost of acquiring new customers reaching unprecedented heights, the ability to track, understand and quantify customer engagement has become essential for businesses.
By focusing on customer engagement, companies can not only enhance customer loyalty and lifetime value but also demonstrate the tangible impact of their marketing and service strategies.
Customer engagement metrics give you the insights and intelligence you need to fine-tune your marketing strategies, ensuring you’re creating experiences that resonate with your target audience.
Table of contents
1. Net Promoter Score (NPS)
Net Promoter Score (NPS) is a widely recognized metric used to gauge customer loyalty and satisfaction. By regularly measuring and analyzing NPS, companies can gain a clear perspective on their customer relationships, identify areas for improvement, and strategically enhance their customer experience. This makes NPS an invaluable tool for businesses aiming to build a loyal customer base and achieve long-term success.
NPS is calculated based on responses to a single, straightforward question:
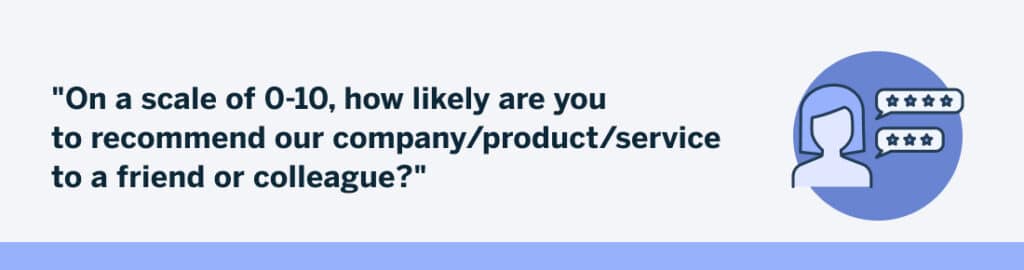
Based on their responses, customers are categorized as Promoters (score 9-10), Passives (score 7-8), or Detractors (score 0-6). The NPS is then derived by subtracting the percentage of Detractors from the percentage of Promoters.
Your NPS serves as a powerful insight into the effectiveness of your customer engagement strategy, shedding light on the general sentiment customers express towards your brand.
A high NPS indicates a healthy, loyal customer base that will keep shopping with your brand and likely refer friends and family to you.
A low NPS, however, is a warning sign you need to pay attention to. It can signal underlying issues in customer satisfaction and loyalty that need to be addressed.
How to improve your NPS
Unlike other customer engagement metrics in this list, it’s hard to directly impact NPS. However, by following these steps, you’ll make improvements across your business that will help it trend in the right direction:
- Understand the “why” behind scores: Dive deep into the feedback from all customer groups (Promoters, Passives, and Detractors) to identify specific areas for improvement.
- Act on customer feedback: Implement changes based on customer suggestions to show that their feedback and opinions are being heard and acted upon.
- Enhance customer service: Focus on training your team to be responsive, empathetic, and solution-oriented, as exceptional customer service can convert Detractors into Promoters. Consider integrating your customer and sales data into your customer service platform so agents can provide a more connected experience.
- Improve product or service quality: Continuously refine your offerings to meet or exceed customer expectations, based on their needs and feedback.
- Regularly measure and analyze NPS: Consistently track and analyze your NPS to understand trends, areas for action, and the impact of the changes you implement.
By implementing these steps, you’ll work towards improving your overall customer satisfaction and experience, which will ultimately be reflected in your NPS.
2. Conversion Rate
Conversion Rate is a key metric that measures the percentage of visitors to your website or platform who take a desired action, such as making a purchase, signing up for a newsletter, or any other goal relevant to your business.
Conversion Rate is calculated with the following equation:
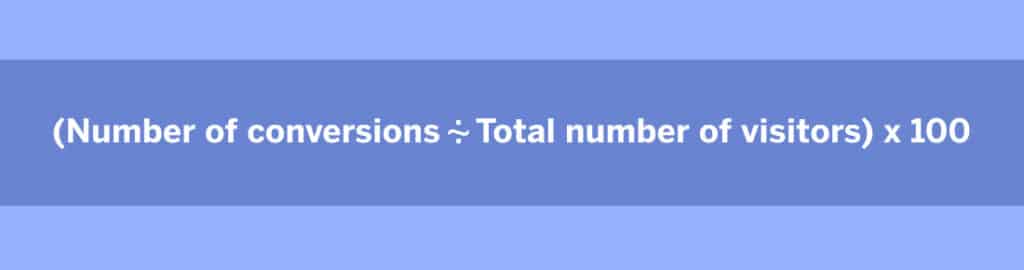
How to analyze your Conversion Rates
Conversion Rate might seem like a “catch-all” metric, but the value of tracking it lies in the insights you can gain from it. This starts with careful analysis to identify what’s working and areas for improvement:
- Contextualize the rate: A Conversion Rate should never be viewed in isolation. Compare it against industry benchmarks, past performance, and expected goals to understand its significance.
- Segment your data: Break down your Conversion Rate by different criteria such as customer demographics, traffic sources, or specific marketing campaigns to help you identify what’s working well and what needs a little more attention.
- Consider the quality of traffic: Not all traffic is equal. High-quality, targeted traffic is more likely to convert than general traffic. Assess the sources and quality of your visitors to better understand your Conversion Rate.
- Look at your funnel stages: Analyze Conversion Rates at different stages of the sales funnel. This can highlight specific areas in the customer journey that need improvement, such as awareness, consideration, or decision stages.
- Factor in external influences: Seasonality, market trends, and promotional events can all impact Conversion Rates. Consider these factors when evaluating performance to get a more accurate reading.
How to optimize Conversion Rates
Improving Conversion Rates is the gold-standard goal for many businesses, because they’re tied so intrinsically to marketing ROI. Optimizing Conversion Rates is often a detailed process underpinned by these five strategies:
- Improve website User Experience (UX): How user-friendly is your website? Does it score well on Google PageSpeed? Is it easy to navigate, and does it render well on mobile?
- Personalize customer experiences: Show your customers you understand them. By tailoring your content and offers to meet the specific needs and preferences of your audience, you can significantly increase engagement and conversions.
- Streamline the checkout process: Retail platforms like Shopify allow full checkout customization on their Plus packages. Simplify the checkout experience to minimize cart abandonment by reducing the number of steps and offering various secure payment options, and include trust signals such as reviews, customer service numbers, and money-back guarantees.
- Use strong, clear CTAs: Ensure that your calls-to-action (CTAs) are prominent and straightforward, guiding customers clearly on what to do next. Effective CTAs are crucial for converting browsing into action.
- Leverage social proof: Display customer testimonials, reviews, and trust badges to build credibility and trust, which can greatly influence decision-making and encourage conversions.
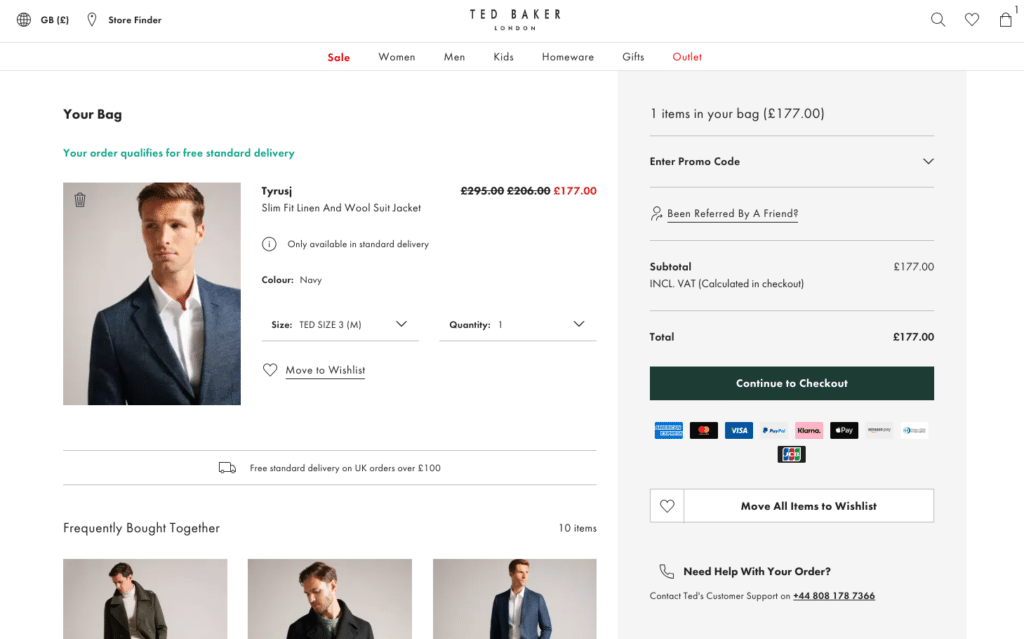
Source: Tedbaker.com
3. Churn Rate
Churn Rate, also known as customer attrition rate, is a critical metric that measures the percentage of customers who stop using a company’s products or services over a specific period. It’s calculated by dividing the number of customers lost during the period by the total number of customers at the start of the period.
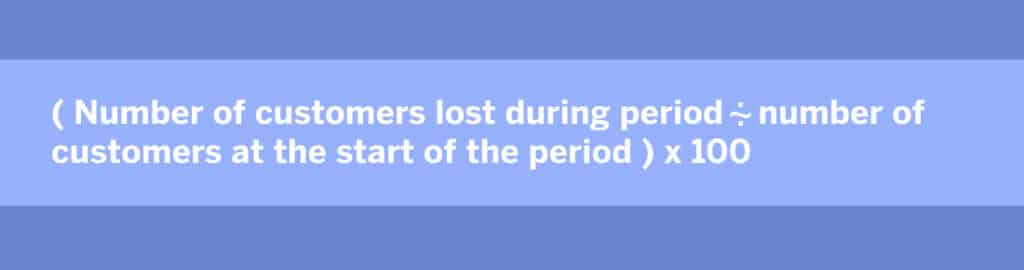
A low Churn Rate suggests strong customer loyalty and satisfaction, which are essential for sustainable growth and profitability. As a result, monitoring and managing Churn Rate is vital for maintaining a healthy customer base and ensuring long-term business success.
A high Churn Rate, on the other hand, often indicates underlying issues such as poor customer service, lack of engagement, or unmet customer needs.
By understanding and tracking your Churn Rate, you’ll help to identify points of friction in your customer journey where you’re losing customers. In a world where it’s 5x more expensive to acquire new customers than to retain old ones, the value of this can’t be overstated.
Tactics to reduce churn and retain customers
Effectively reducing churn and retaining customers is vital for maintaining a healthy and thriving business. Let’s take a look at four strategies proven to help reduce churn:
- Enhance customer service: Integrate customer service with your key marketing channels. This integration gives customer service representatives access to vital customer and sales data, helping them to provide a seamless, connected experience that meets customer needs effectively.
- Offer personalized experiences: Personalization fosters a deeper connection and increases customer loyalty. As a result, tailoring your content to each customer’s unique needs and preferences can help keep them engaged and coming back.
- Gather and act on feedback: Regularly collect and respond to customer feedback. Showing that you value and act on their input can significantly boost customer satisfaction and reduce churn.
- Implement a customer loyalty program: Develop a loyalty program that rewards and incentivizes repeat business. This encourages customers to continue engaging with your brand.
Read next: 20 Retail Brands with the Best Customer Loyalty Programs
4. Customer Lifetime Value (CLV)
Customer Lifetime Value (CLV) is a critical metric in business that represents the total revenue a company can expect from a single customer throughout their entire relationship. It measures the financial value of each customer, factoring in their revenue contribution over time minus the costs associated with acquiring and serving them.
The significance of CLV lies in its long-term perspective. Unlike metrics that focus on immediate sales or transactions like Conversion Rate, CLV provides insight into the enduring value a customer brings.
How to Calculate Customer Lifetime Value
The basic formula for CLV is:
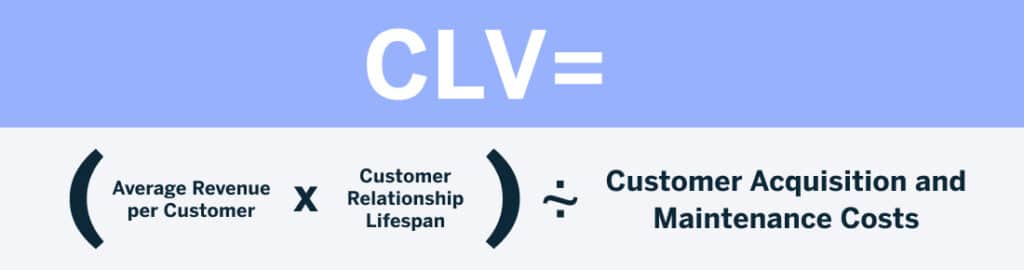
Let’s take a look at the elements in this calculation:
- Average revenue per customer: This is typically calculated by dividing the total revenue over a certain period by the number of customers during that period.
- Customer relationship lifespan: This is an estimate of the average duration a customer continues to purchase from your business.
- Customer acquisition and maintenance costs: These are the costs associated with acquiring a new customer and maintaining relationships with existing ones.
Across multiple online and offline channels, identifying your true CLV can become a challenge. Modern customer engagement platforms like SAP Emarsys significantly simplify this process. These platforms can automatically calculate CLV by integrating sales, marketing, and customer service data. This automation provides a more dynamic and accurate picture of CLV, as it incorporates real-time data and customer interactions.
How to improve Customer Lifetime Value
Maximizing Customer Lifetime Value (CLV) is essential for sustained business growth. Here are five proven strategies to enhance CLV:
- Offer exceptional customer service: Superior customer service with response and empathetic interactions foster loyalty and repeat business, directly contributing to an increased CLV.
- Implement a loyalty program: Rewarding repeat customers with a well-structured loyalty program encourages ongoing purchases and referrals, significantly impacting CLV.
- Upsell and cross-sell effectively: Introducing customers to complementary or premium products increases the average transaction value, thereby boosting CLV.
- Focus on high-value customers: Identify and prioritize your most profitable customer segments, tailoring your efforts to meet their specific needs and increase their engagement and spending.
- Use data analytics for personalization: Leverage customer data to create personalized experiences and targeted marketing campaigns, which can significantly enhance customer satisfaction and loyalty.
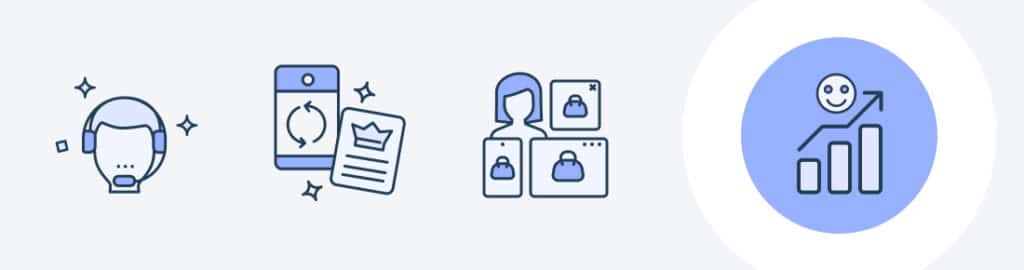
5. Google Analytics Event Count
Google Analytics Event Count is a metric that quantifies the number of specific interactions, or “events,” that users have with a website or app. These events can include a wide range of activities, such as clicks on links, video plays, form submissions, and downloads.
With the transition to Google Analytics 4 (GA4), the concept of events has become even more central to how user interactions are tracked.
Unlike its predecessor, GA4 uses an event-based model for data collection, offering more flexibility and a deeper level of insight into user behavior. In GA4, virtually any user interaction can be tracked as an event, providing a more comprehensive understanding of how users engage with your site or app.
The importance of monitoring Event Count in GA4 lies in its ability to provide detailed insights into user behavior. By analyzing these events, businesses can identify which aspects of their site or app are most engaging, which features are being utilized, and where potential issues may lie.
This data is invaluable for optimizing user experience, improving site functionality, and ultimately driving conversions.
Analyzing GA Event Count for user behavior insights
Effectively analyzing the Event Count in Google Analytics (GA), especially with the advanced capabilities of GA4, can provide deep insights into user behavior.
Here’s how to leverage this data:
- Identify key events: Determine which events are most critical for your business objectives. This could be page views, button clicks, form submissions, or product purchases.
- Segment event data: Break down the event data by various dimensions such as user demographics, traffic sources, device used, or time of day. This segmentation helps in understanding the different behaviors of various user groups.
- Monitor event trends over time: Observe how event counts change over time. Trends can indicate shifts in user behavior, the impact of marketing campaigns, or emerging issues with your website or app.
- Compare events with conversion metrics: Align event data with conversion metrics to see how specific interactions contribute to your ultimate business goals. Understanding this correlation is key to optimizing for conversions.
- Utilize Event Flow reports: GA4 offers Event Flow reports that visually represent the path users take through your site or app, marked by the events they trigger. Analyzing these paths can reveal common user journeys and potential roadblocks.
- Set up Event Goals: In GA4, you can set up specific events as goals. Tracking these goals helps in quantifying how users are interacting with your website.
- Leverage User Exploration reports: Use GA4’s User Exploration feature to conduct an in-depth analysis of user behavior. This tool allows for a more customized analysis based on specific events and user attributes.
By thoroughly analyzing Event Count data in GA4, businesses can gain valuable insights into user behavior, enabling them to make informed decisions for enhancing user experience, refining marketing strategies, and ultimately driving better business outcomes.
Leveraging event data for improved engagement
Effectively using event data from Google Analytics 4 (GA4) can significantly enhance customer engagement. Here are the four most crucial steps:
- Tailor user experience based on interaction patterns: Use insights from event data to refine your website or app’s user experience. Focus on simplifying navigation, enhancing key features, and removing barriers based on how users interact with your website.
- Personalize content and offers: Segment your audience according to their event interactions and tailor content and offers to match their preferences and behaviors.
- Optimize conversion funnels: Analyze events within your conversion funnel to identify drop-off points. Streamlining these areas, such as the checkout process, can significantly improve the user journey and conversion rates.
- Measure impact of changes: Continuously monitor how changes based on event data affect user behavior. This ongoing analysis is key to understanding the effectiveness of your optimizations and guiding further improvements.
By focusing on these key areas, businesses can effectively leverage GA4 event data to create a more engaging, personalized, and efficient experience for their users, driving enhanced engagement and conversions.
6. Average Order Value (AOV)
Average Order Value (AOV) is a key ecommerce metric that measures the average amount spent each time a customer places an order on a website or an app. It’s calculated by dividing the total revenue by the number of orders over a given period.
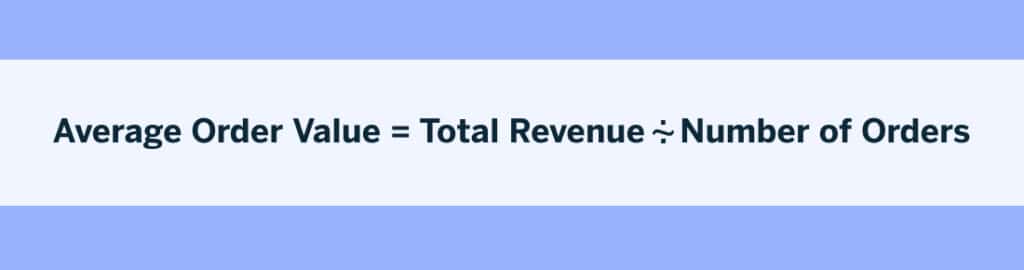
AOV is an essential indicator not just of the perceived value of your products, but also how effective your customer engagement strategy is at getting relevant products in front of each customer. By tracking AOV, companies can gauge how much their customers are willing to spend per purchase, providing insight into their spending behavior.
Techniques to increase Average Order Value
Increasing the AOV directly impacts revenue growth, even without a corresponding increase in traffic or customer count. This makes it a crucial metric for businesses aiming to maximize revenue efficiently.
Tactics to increase Average Order Value include:
- Upselling: Encourage customers to purchase a higher-end version of the chosen product. Highlight the benefits and value of the premium option.
- Cross-selling: Suggest related products or accessories that complement the customer’s initial choice. This not only adds value to their purchase but also increases the order total.
- Personalized recommendations: Use customer data to provide personalized product recommendations, increasing the likelihood of additional purchases.
- Volume discounts: Offer discounts on bulk purchases to incentivize customers to buy more. For example, a discount on buying three or more items.
- Bundle products: Create product bundles that offer a better value than purchasing items separately. This encourages customers to spend more to get the “bundle deal.”
- Free shipping threshold: Set a minimum purchase amount for free shipping that’s slightly above your AOV. This can motivate customers to add more items to their cart to qualify for free shipping.
- Time-limited offers: Create urgency with time-limited offers or promotions, prompting customers to make larger purchases to take advantage of the deal.
- Loyalty programs: Implement a loyalty program that rewards customers based on their spending. This can encourage repeat purchases and higher order values over time.
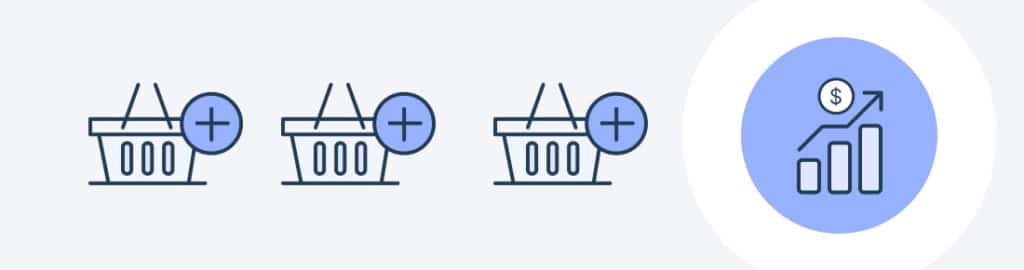
7. Customer Retention Rate (CRR)
Customer Retention Rate (CRR) is a crucial metric that measures the percentage of customers a company retains over a specific period.
The formula for calculating Customer Retention Rate (CRR) is as follows:
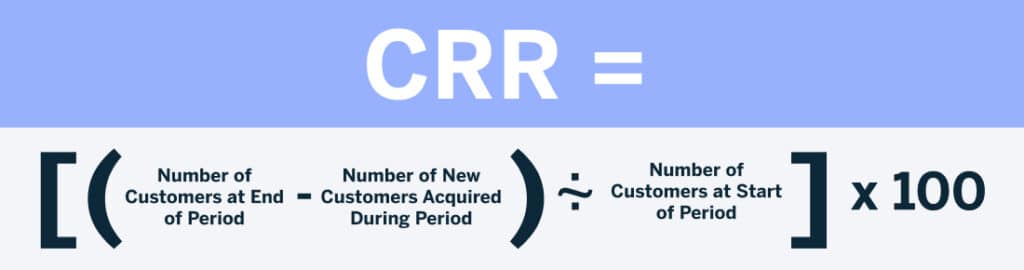
This formula calculates what percentage of customers a business has managed to retain over a certain period, without considering any new customers acquired during that time, giving you a baseline for the performance of your customer retention strategies.
The significance of Customer Retention Rate lies in its focus on the long-term relationships a business maintains with its customers.
High retention rates are indicative of customer satisfaction, loyalty, and the value a business provides to its existing customer base.
In contrast, low retention rates can signal dissatisfaction or disengagement, prompting a need for improved customer experiences or value propositions.
8. Social Media Engagement Metrics
Social Media Engagement Metrics are key indicators that reflect how audiences interact with a brand’s content on various social media platforms, such as likes, comments, shares, retweets, mentions, and follower growth.
These metrics are essential in measuring the effectiveness of a brand’s social media presence and understanding the impact of its content on the audience.
To gauge the impact of your customer engagement strategies, track changes in the following metrics:
- Likes and Reactions: These are initial indicators of how well your content is resonating with your target audience. An increase in likes and reactions suggests that the content is appealing to your audience. Brands should aim for a steady growth in these metrics as a sign of consistent audience approval.
- Comments: Comments reflect deeper engagement and can provide qualitative feedback. An increase in comments often indicates higher interest and interaction with your content. Brands should look for more frequent and meaningful conversations in this metric.
- Shares/Reposts: These metrics are a sign of endorsement from your audience. More shares and reposts mean your content is compelling enough for users to share with their network, expanding your audience and influence.
- Reach and Impressions: Tied to other metrics like reactions, shares, and reposts, reach and impressions measure the extent of your content’s visibility. Higher reach and impressions show your content is capturing a wider audience, which is vital for top-of-funnel brand awareness.
- Click-through Rate (CTR): CTR measures how effectively your content prompts action. An improving CTR indicates that your calls-to-action are resonating and driving traffic, a key goal for most digital campaigns.
- Engagement Rate: This is a catch-all metric that provides a heads-up view of how engaging your content is. An upward trend in engagement rate is an indicator of deepening audience relationships with your brand.
- Follower Growth Rate: This rate tracks how quickly your audience is expanding. Accelerated follower growth can be a sign of effective content strategy and brand popularity.
- Social mentions: Coupled with sentiment analysis, social mentions give you invaluable insight into the effectiveness of your overall customer experience.
- Video views and watch time: This doesn’t just show how many views you’re getting, but also how long viewers are staying engaged. Increasing watch time is a sign that your video content strategy is working.
By tracking these metrics, you’ll help your team to identify what’s working, what isn’t, and how you can improve your strategy to better engage your audience across the customer lifecycle.
Building a Metrics-Driven Strategy
In the world of modern marketing, where multiple channels are at play, aligning your business objectives with a metrics-driven strategy is essential to success. By setting and monitoring Key Performance Indicators (KPIs), you’ll help track progress towards your customer engagement goals and make informed decisions on the effectiveness of your strategy.
To align your key customer engagement metrics with your overall business objectives, follow these 7 steps:
- Identify Core Business Objectives: Start by clearly defining your business objectives. Whether it’s increasing revenue, enhancing customer satisfaction, or expanding market share, your objectives should guide the choice of metrics.
- Select Relevant Metrics: Choose metrics that directly reflect the progress of these objectives. For instance, if a core goal for your business is to increase Monthly Recurring Revenue (MRR), Conversion Rate, Average Order Value, Churn, and Customer Lifetime Value are relevant metrics you should consider tracking.
- Set Specific, Measurable Targets: Establish specific targets for each KPI. These targets should be measurable, achievable, relevant, and time-bound, aligning with the SMART criteria.
- Monitor and Analyze Regularly: Consistently track these metrics over time. Regular analysis helps in understanding trends, identifying areas for improvement, and gauging the effectiveness of your strategies.
- Adapt and Evolve: No customer engagement strategy is “set and forget.” As a result, be prepared to adapt your strategy based on metric performance. If certain KPIs aren’t meeting targets, investigate underlying causes and adjust your tactics accordingly.
- Integrate Across Departments: Ensure that these KPIs are integrated into all relevant departments. A unified approach ensures that everyone is aligned and working towards the same business objectives.
- Leverage Customer Engagement Platforms: Use digital tools and analytics platforms for real-time tracking and deeper insights. Platforms like SAP Emarsys can integrate your channels and data, giving you a sophisticated view of performance across your key touchpoints.
Use Emarsys for Effective and Accurate Metric Management
From unifying sales, product, and transactional data to enabling 1:1 personalization and orchestrating sophisticated cross-channel campaigns — tens of thousands of marketers from the world’s most innovative brands choose the SAP Emarsys Customer Engagement platform to drive revenue and accelerate business outcomes.
Paired with built-in AI-powered analytics, Emarsys gives marketers the intelligence to know what’s working, and the insights to know what will work next, helping you to drive the revenue metrics that matter.